Colors
CSS Colors are specified using with , , ,,- color name
- Red
- Blue
- RGB
- Hex
- Hsl
- RGBA
Background
The CSS background properties are used to define the background effects for elements.- background-color
- background-image
- background-repeat
- background-attachment
- background-position
<html>
<head>
<style>
h1 {
background-color: lightgreen;
}
div {
background-color: lightblue;
}
p {
background-color: yellow;
}
body{
background-image: url("https://images.pexels.com/photos/956981/milky-way-starry-sky-night-sky-star-956981.jpeg?auto=compress&cs=tinysrgb&h=350");
background-repeat: repeat-x;
background-position: top;
background-attachment: fixed;
}
</style>
</head>
<body>
<h1>CSS background-color example!</h1>
<div>
This is a text inside a div element.
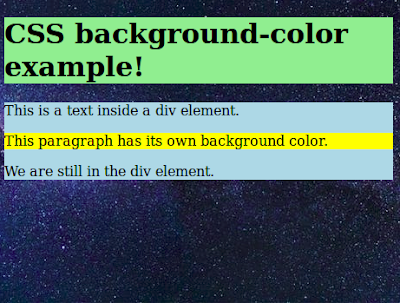
We are still in the div element.
</div>
</body>
</html>
Border
- CSS has 10 boredrs style
- dotted - Defines a dotted border
- dashed - Defines a dashed border
- solid - Defines a solid border
- double - Defines a double border
- groove - Defines a 3D grooved border. The effect depends on the border-color value
- ridge - Defines a 3D ridged border. The effect depends on the border-color value
- inset - Defines a 3D inset border. The effect depends on the border-color value
- outset - Defines a 3D outset border. The effect depends on the border-color value
- none - Defines no border
- hidden - Defines a hidden border
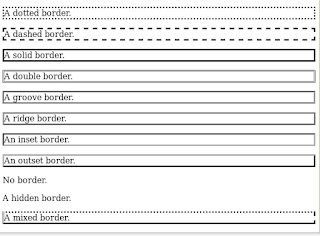
<html>
<head>
<style>
p.dotted {border-style: dotted;}
p.dashed {border-style: dashed;}
p.solid {border-style: solid;}
p.double {border-style: double;}
p.groove {border-style: groove;}
p.ridge {border-style: ridge;}
p.inset {border-style: inset;}
p.outset {border-style: outset;}
p.none {border-style: none;}
p.hidden {border-style: hidden;}
p.mix {border-style: dotted dashed solid double;}
</style>
</head>
<body>
<p class="dotted">A dotted border.</p>
<p class="dashed">A dashed border.</p>
<p class="solid">A solid border.</p>
<p class="double">A double border.</p>
<p class="groove">A groove border.</p>
<p class="ridge">A ridge border.</p>
<p class="inset">An inset border.</p>
<p class="outset">An outset border.</p>
<p class="none">No border.</p>
<p class="hidden">A hidden border.</p>
<p class="mix">A mixed border.</p>
</body>
</html>
Border-style
- If the border-style property has four values
- border-style: dotted solid double dashed
- top border is dotted
- right border is solid
- bottom border is double
- left border is dashe
- If the border-style property has three values
- border-style: dotted solid double
- top border is dotted
- right and left borders are solid
- bottom border is double
- If the border-style property has two values
- If the border-style property has two values
- top and bottom borders are dotted
- right and left borders are solid
- If the border-style property has one value
- border-style: dotted
- all four borders are dotted
Margin
- CSS has properties for specifying the margin for each side of an element
- margin-top
- margin-right
- margin-bottom
- margin-left
- All the margin properties can have the following values
- auto - the browser calculates the margin
- length - specifies a margin in px, pt, cm, etc.
- % - specifies a margin in % of the width of the containing element
- inherit - specifies that the margin should be inherited from the parent element
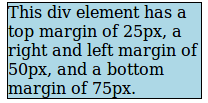
<html>
<head>
<style>
div {
border: 1px solid black;
margin: 25px 50px 75px;
background-color: lightblue;
}
</style>
</head>
<body>
<div>This div element has a top margin of 25px, a right and left margin of 50px, and a bottom margin of 75px.</div>
</body>
</html>
Text Formating
- Text Color
- Text Alignment
- Text Decoration
- Text Transformation
- Text Indentation
- Letter Spacing
- Line Height
- Text Direction
- Word Spacing
- Text Shadow
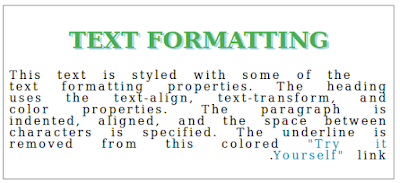
<html>
<head>
<style>
div {
border: 1px solid gray;
padding: 8px;
}
h1 {
text-align: center;
text-transform: uppercase;
color: #4CAF50;
text-shadow:9px 2px lightblue;
}
p {
text-indent: 50px;
text-align: justify;
letter-spacing: 3px;
word-spacing: 10px;
direction: rtl;
line-height: 1;
letter-spacing: 3px;
}
a {
text-decoration: none;
color: #008CBA;
}
</style>
</head>
<body>
<div>
<h1>text formatting</h1>
<p>This text is styled with some of the text formatting properties. The heading uses the text-align, text-transform, and color properties.
The paragraph is indented, aligned, and the space between characters is specified. The underline is removed from this colored
<a target="_blank" href="tryit.asp?filename=trycss_text">"Try it Yourself"</a> link.</p>
</div>
</body>
</html>
Table
- Table Borders
- Collapse Table Borders
- Table Width and Height
- Horizontal Alignment
- Vertical Alignment
- Table Padding
- Horizontal Dividers
- Hoverable Table
- Table Color
<html>
<head>
<style>
table {
border-collapse: collapse;
width: 100%;
}
th, td {
text-align: left;
padding: 8px;
border-bottom: 6px solid #ddd;
}
tr:nth-child(even){background-color: #f2f2f2}
th {
background-color: #4CAF50;
color: white;
}
tr:hover {background-color: #f5f5f5;}
td {
height: 30px;
vertical-align: middle;
}
</style>
</head>
<body>
<h2>Colored Table Header</h2>
<table>
<tr>
<th>Firstname</th>
<th>Lastname</th>
<th>Savings</th>
</tr>
<tr>
<td>Peter</td>
<td>Griffin</td>
<td>$100</td>
</tr>
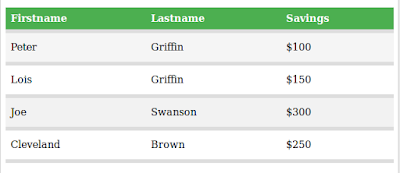
<td>Lois</td>
<td>Griffin</td>
<td>$150</td>
</tr>
<tr>
<td>Joe</td>
<td>Swanson</td>
<td>$300</td>
</tr>
<tr>
<td>Cleveland</td>
<td>Brown</td>
<td>$250</td>
</tr>
</table>
</body>
</html>
Nvigation Bar
- Having easy-to-use navigation is important for any web site.
<html>
<head>
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<style>
body {margin: 0;}
ul.topnav {
list-style-type: none;
margin: 0;
padding: 0;
overflow: hidden;
background-color: #333;
}
ul.topnav li {float: left;}
ul.topnav li a {
display: block;
color: white;
text-align: center;
padding: 14px 16px;
text-decoration: none;
}
ul.topnav li a:hover:not(.active) {background-color: #111;}
ul.topnav li a.active {background-color: #4CAF50;}
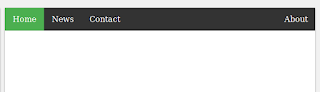
ul.topnav li.right {float: right;}
@media screen and (max-width: 600px) {
ul.topnav li.right,
ul.topnav li {float: none;}
}
</style>
</head>
<body>
<ul class="topnav">
<li><a class="active" href="#home">Home</a></li>
<li><a href="#news">News</a></li>
<li><a href="#contact">Contact</a></li>
<li class="right"><a href="#about">About</a></li>
</ul>
</body>
</html>
Refreance : https://www.w3schools.com/css/default.asp
No comments:
Post a Comment